image compression using K-means clustering
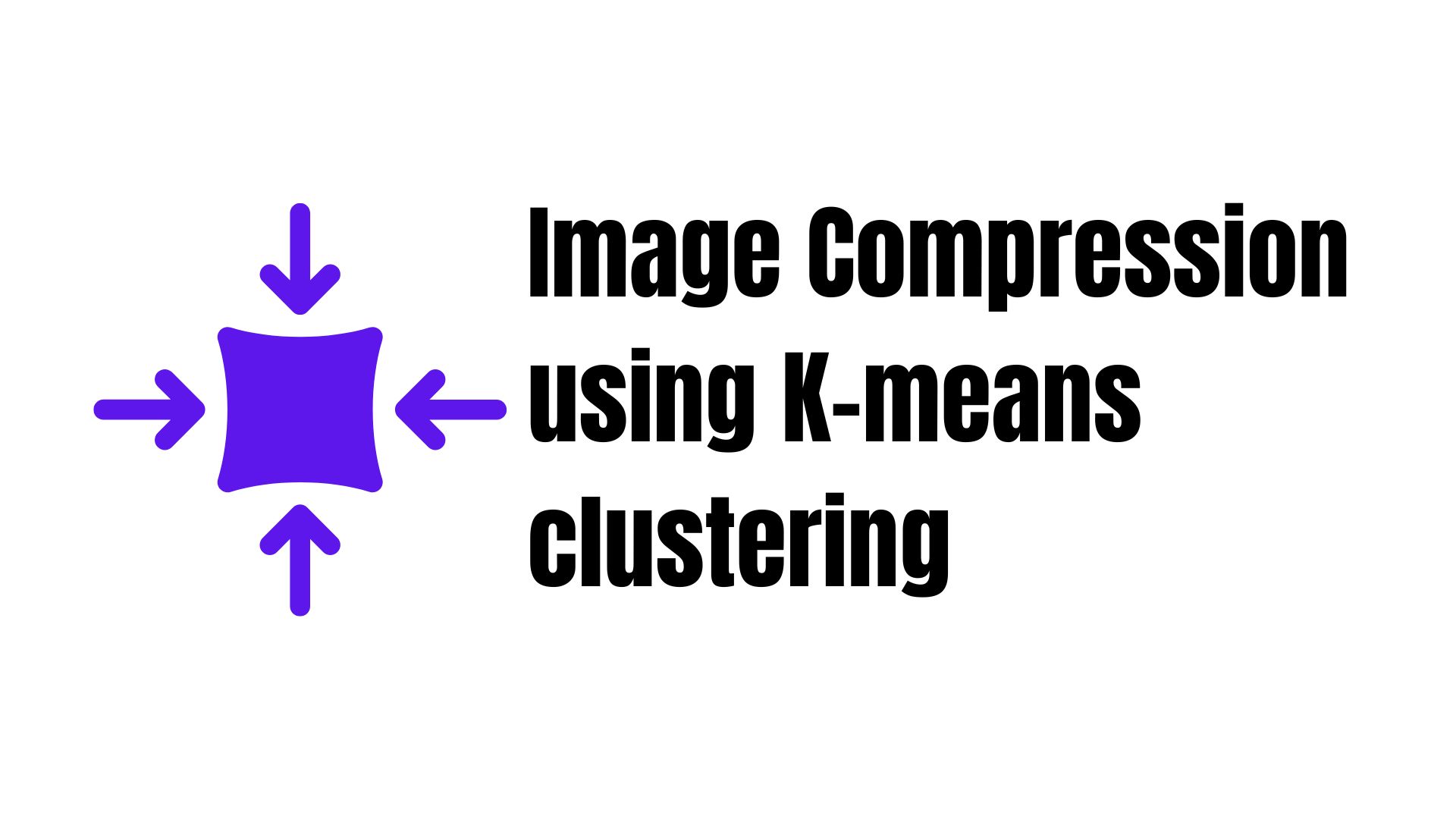
To deeply understand the concept of image compression using K-means clustering, let’s break it down into its core elements, step by step:
1. RGB Encoding in an Image
- Image Representation:
- In digital images, each pixel is represented using the RGB color model, where:
- Red (R), Green (G), and Blue (B) intensities are combined to form a specific color.
- Each component (R, G, B) is an 8-bit unsigned integer ranging from 0 to 255.
- For example:
- A pixel with RGB values (255, 0, 0) is pure red.
- A pixel with RGB values (0, 255, 0) is pure green.
- A pixel with RGB values (0, 0, 255) is pure blue.
- This means each pixel requires 3 bytes (24 bits) to store its color information.
- In digital images, each pixel is represented using the RGB color model, where:
2. Motivation for Compression
- Problem: A typical image might have thousands or even millions of unique colors, leading to large storage requirements.
- Solution: Reduce the number of colors used to represent the image while maintaining a visually acceptable quality.
- Instead of storing the exact RGB values for every pixel, we can:
- Cluster the colors into a small number of representative colors.
- Replace each pixel’s color with the nearest representative color in the clustered palette.
3. The Role of K-means Clustering
- K-means is a clustering algorithm that groups data points into kk clusters. Here’s how it applies to image compression:
- Data Preparation:
- Treat every pixel in the image as a 3-dimensional data point (R,G,B)(R, G, B).
- For an image with h×wh \times w pixels, you’ll have h×wh \times w data points in the RGB space.
- Clustering:
- Use the K-means algorithm to group these data points into kk clusters (e.g., k=16k = 16 for 16 colors).
- Each cluster is represented by its centroid, which becomes one of the 16 representative colors.
- Compression:
- Replace each pixel’s RGB value with the RGB value of the nearest cluster centroid.
- Representation:
- Instead of storing the original RGB values, store:
- The 16 centroids (each requiring 24 bits of storage).
- For each pixel, an index indicating which of the 16 centroids it belongs to (requires only 4 bits per pixel, as 24=162^4 = 16).
- Instead of storing the original RGB values, store:
- Data Preparation:
4. Step-by-Step Explanation of Image Compression
- Input:
- A high-resolution image with h×wh \times w pixels.
- Thousands of unique RGB color combinations.
- Apply K-means:
- Treat each pixel’s color (R,G,B)(R, G, B) as a point in 3D space.
- Run K-means to group all pixels into 16 clusters:
- Centroids: The mean RGB value of all pixels in a cluster.
- Clusters: Groups of pixels that are closest to a particular centroid.
- Compress the Image:
- Replace each pixel’s RGB value with the RGB value of the nearest centroid.
- The image now uses only 16 colors instead of thousands.
- Storage:
- Instead of storing the full RGB value for each pixel:
- Store the 16 RGB centroids (16 × 24 bits).
- Store a 4-bit index for each pixel (representing the centroid it belongs to).
- Instead of storing the full RGB value for each pixel:
5. Benefits and Trade-offs
- Benefits:
- Significantly reduces the image size while retaining an acceptable quality.
- Efficient representation: Storing indices (4 bits per pixel) instead of full RGB values (24 bits per pixel) drastically reduces storage requirements.
- Simplifies image processing tasks by reducing color complexity.
- Trade-offs:
- Loss of fine details: Some color gradations and subtle differences are lost in the compressed image.
- Visual artifacts: The compressed image might have a blocky or posterized appearance if the number of clusters (kk) is too low.
6. Practical Implementation Workflow
- Load the Image:
- Read the image into a matrix of pixel values.
- Reshape the matrix into a list of RGB values for each pixel.
- Run K-means:
- Use a machine learning library (e.g., Python’s
scikit-learn
) to perform K-means clustering on the RGB values. - Specify k=16k = 16 to find 16 representative colors.
- Use a machine learning library (e.g., Python’s
- Map Pixels to Centroids:
- Replace each pixel’s color with the nearest centroid’s RGB value.
- Save the Compressed Image:
- Reconstruct the image using the compressed representation and save it.
7. Visualizing the Compression
- Original Image: Full range of colors, high storage.
- Compressed Image:
- Limited to 16 colors.
- Slight loss of detail but visually similar to the original image.
- Drastically reduced file size.
By understanding these steps, you can see how K-means clustering leverages mathematical optimization to balance image quality and storage efficiency.